List
특징 : 인덱스를 기반으로 자료를 순차적으로
일반 배열과 List 비교하는것
먼저 strArray는 데이터 크기를 정하지만
strList는 처음에는 공간이 없다가 컴퓨터가 자리를 만들어준다.
출력은 이렇게 됨
add 메서드
arrayList에 마지막에 add를 한다.
strList.add(2,"C"); 로 인해서 C가D자리를 세치기해서 D는 공간이 더 생긴다.
List 계역의 특징 :
마지막에 데이터를 삽입/삭제 할 때는 효율적으로 동작
중간에 데이터를 삽입/삭제 할 때는 비효율적으로 동작
remove 메서드는 괄호에 인덱스 번호를 넣으면 삭제가 된다!!!!!
파란줄 해석 방법 !!!!!! 꼭 알아야함
String java.util.ArrayList.remove(int index)
파악 방법
string 반환타입
어디 선언된다 java.util.ArrayList.
사용할 메서드는 remove
매개변수는 int 이며 이름은 index 이다
해석하는거 계속 연습하기
intList 1~10 데이터를 넣고 출력을 하시오
주석으로 한것은 순서대로 하고 for문을 사용해서 반복으로 표현함
메서드 사이즈 하는것은 size() 로 쓴다
향상된 for문
============================================================
활용
문제
KTX 승차권 관리 프로그램 개발하려고 합니다.
승차권을 구매할때 구매자(customer), 이름(name), 연락처(tel), 좌석번호(seat) 입력합니다.
구매자의 정보를 저장하려고 합니다.
오른쪽에 두명의 구매자 정보를 참고해서 승차권 관리 프로그램을 개발하세요~!
구매자1 | 구매자2 | |
이름 | 홍길동 | 고영희 |
연락처 | 010-1473-3698 | 010-1598-7894 |
좌석번호 | 32 | 55 |
1. 먼저 class를 만들어서 해당되는 멤버변수와 메서드를 입력합니다.
*생성자는 간단하게 출력하는 방법
이걸로 간단하게 생성자 메서드를 만들수있다.
멤버변수가 있으므로 get, set 메서드 만들어주기!!!!!!!!!!!!!!!!!!!
ArrayList괄호에 저장해야하는것을 넣는다 여기에는 Customer을 넣느나~~
복습 다시 하기 차근차근~~~~
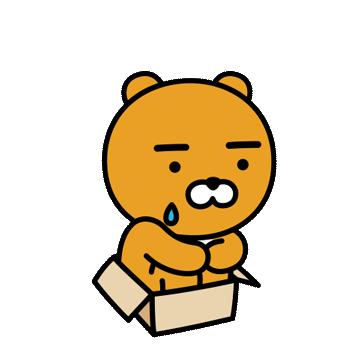
두 구매자 정보를 저장완료
오버라이딩으로 출력하기
==========================================================================
밑에꺼 다시 공부하기!!!!!!!!!!!!
package chapter17;
public class Customer {
private String name;
private String tel;
private int seat;
//멤버 변수가 있으면 get ,set 메서드 필요함
// 생성자 만들어줌
// super 우리 눈에 안보이지만 extends Object
public Customer(String name, String tel, int seat) {
this.name = name;
this.tel = tel;
this.seat = seat;
}
//Object 클래스가 물려주는 메서드 중에 equals 메서드가 있음
// equals 메서드의 용도-> 이객체와 다른 객체를 비교하기 위한 용도
@Override
public boolean equals(Object obj) {
Customer otherCustomer = (Customer) obj;
String otherName = otherCustomer.getName();
String otherTel = otherCustomer.getTel();
int otherSeat = otherCustomer.getSeat();
boolean nameSame = name.equals(otherName);
boolean telSame = tel.equals(otherTel);
boolean seatSame = seat == otherSeat;
return nameSame && telSame && seatSame;
}
@Override
public String toString() {
// 이 객체가 가지고 있는 멤버변수를 보기 좋게 문자열로 구성해서 return
return "name ="+name+", tel =" +tel +", seat = "+seat;
}
// get, set 메서드
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getTel() {
return tel;
}
public void setTel(String tel) {
this.tel = tel;
}
public int getSeat() {
return seat;
}
public void setSeat(int seat) {
this.seat = seat;
}
}
package chapter17;
import java.util.ArrayList;
public class Ex04 {
public static void main(String[] args) {
// TODO Auto-generated method stub
Customer c1 = new Customer("홍길동", "010-1473-3698", 32);
Customer c2 = new Customer("고영희", "010-1598-7894", 55);
//객체를 Sysout으로 출력하면 객체가 가지고 있는 toString메서드가 호출됨.
// 아래 두개 같음
System.out.println(c1);
System.out.println(c2);
ArrayList<Customer> list = new ArrayList<Customer>();
list.add(c1);
list.add(c2);
//list에 저장된 각 객체가 오버라이딩 된 tostring메서드를 가지고 있으므로
// ArrayList를 출력하면 각 객체가 가지고 있는 tostring메서드가 호출되
// 보기좋게 출력됨
System.out.println(list);
// c1과 c2가 같은 객체인지 비교해보세요.
// 같은 객체인지 비교한다 -> 같은 정보를 가지고 있는지 비교한다.
// 두 객체가 같다면 "같다' 를 출력
// 두 객체가 다르다면 "다르다"를 출력
// if(c1 == c2) {
// System.out.println("같다");
// } else {
// System.out.println("다르다");
// }
// 위에꺼 잘못됨
// 당연히 메모리주소가 다르므로 다르다고 나옴 c1과 c2 의 메모리주소가 같나요? 라고 물어보는거임 의도와 다름
// String c1Name = c1.getName();
// String c2Name = c2.getName();
//
// String c1Tel = c1.getTel();
// String c2Tel = c2.getTel();
//
// int c1Seat = c1.getSeat();
// int c2Seat = c2.getSeat();
//
// if(c1Name.equals(c2Name) && c1Tel.equals(c2Tel) && c1Seat == c2Seat ){
// System.out.println("같다");
// }else {
// System.out.println("다르다");
// }
if(c1.equals(c2)) {
System.out.println("같다");
} else {
System.out.println("다르다");
}
}
}
package chapter17;
import java.util.ArrayList;
public class Ktx2 {
public void printAllCustomer(ArrayList<Customer> list) {
for(Customer nthCustomer : customerList) {
System.out.println("이름 : " + nthCustomer.getName());
System.out.println("연락처 : " + nthCustomer.getTel());
System.out.println("좌석번호 : " + nthCustomer.getSeat());
}
}
public static void main(String[] args) {
// TODO Auto-generated method stub
// 승차권을 구매한 구매자의 정보를 저장
ArrayList<Customer> customerList = new ArrayList<Customer>();
Customer c1 = new Customer("홍길동", "010-1473-3698", 32);
Customer c2 = new Customer("고영희", "010-1598-7894", 55);
customerList.add(c1);
customerList.add(c2);
System.out.println(customerList);
//customerList에 저장된 두 구매자의 정보를 출력하세요
//향상된 for문
Ex03 ex03 = new Ex03();
ex03.printAllCustomer(customerList);
Customer c3 = new Customer("김영수", "010-7536-9514", 17);
customerList.add(0, c3);
ex03.printAllCustomer(customerList);
Stirng removeItem = customerList.remove(1);
System.out.println(customerList);
System.out.println("삭제된 데이터 -> " + removeItem);
int n = 0;
for(Cutsomer c : customerList) {
if(c.getName().equals("홍길동")) {
customerList.remove(n);
}
n++;
}
for(int n =0; n<customerList.size(); n++) {
Customer c = customerList.get(n);
if(c.getName().equals("홍길동")) {
customerList.remove(n);
}
n++;
}
};
}
package chapter17;
import java.util.ArrayList;
public class Ex01 {
public static void main(String[] args) {
// TODO Auto-generated method stub
ArrayList<String> strList = new ArrayList<String>();
strList.add("A");
strList.add("B");
System.out.println(strList);
strList.add("D");
System.out.println(strList);
// 지정한 index부터 마지막 데이터까지 한 칸씩 뒤로 미루고
// 모두 미뤄진 후에 지정한 index에 데이터가 들어감
strList.add(2, "C");
System.out.println(strList);
String str = strList.get(0);
System.out.println("0번 인덱스 데이터 : " + str);
str = strList.get(1);
System.out.println("1번 인덱스 데이터 : " + str);
str = strList.get(2);
System.out.println("2번 인덱스 데이터 : " + str);
str = strList.get(3);
System.out.println("3번 인덱스 데이터 : " + str);
//remove 안에 숫자는 인덱스 숫자 넣는다.
String removedItem = strList.remove(0);
System.out.println(strList);
System.out.println("삭제한 데이터 ->" + removedItem);
}
}
'코딩 > java (백엔드공부)' 카테고리의 다른 글
java 조건문 기초 #2 (0) | 2022.04.19 |
---|---|
java 완전 기초 복습 #1 (0) | 2022.04.19 |
java chatper17 컬렉션 프레임 워크 (0) | 2022.04.18 |
java chater16 제네릭스(Generics) = 지네릭스 (0) | 2022.04.18 |
java chaper16 레퍼클래스(wapper class), 오토박싱, 언박싱 (0) | 2022.04.18 |